作成方法
Apex REST API コールアウトユーティリティ(Apex REST API Callouts Utility)を作成するには、以下の手順を参考にしてください。
-
Apex クラスの作成: 新しい Apex クラスを作成し、Callout ユーティリティのメソッドを含めます。例えば、「APIUtility」という名前のクラスを作成します。
-
HTTP リクエストの作成: Callout メソッドを作成して、HTTP リクエストを構築します。
public class APIUtility {
public static HttpResponse sendGetRequest(String endpoint) {
HttpRequest request = new HttpRequest();
request.setEndpoint(endpoint);
request.setMethod('GET');
// ヘッダーの設定などが必要な場合は追加してください
Http http = new Http();
HttpResponse response = http.send(request);
return response;
}
}
- HTTP レスポンスの処理: レスポンスを受け取るメソッドを作成し、レスポンスを処理します。
public class APIUtility {
public static HttpResponse sendGetRequest(String endpoint) {
// ...
HttpResponse response = http.send(request);
return response;
}
public static String processResponse(HttpResponse response) {
if (response.getStatusCode() == 200) {
return response.getBody();
} else {
// エラー処理などを行う場合は追加してください
return null;
}
}
}
- ユーザーコードでの使用: Callout ユーティリティを使用するために、適切なエンドポイントを指定してメソッドを呼び出します。
String endpoint = 'https://api.example.com/data';
HttpResponse response = APIUtility.sendGetRequest(endpoint);
String responseBody = APIUtility.processResponse(response);
// responseBodyを適切に処理するコードを追加してください
- 上記の例では、GET リクエストの送信とレスポンスの処理を行っていますが、必要に応じて POST や PUT などの他の HTTP メソッドをサポートするメソッドを追加することもできます。
またヘッダーやパラメータを指定することができます。
public class APIUtility{
/**リクエストメソッド */
private enum RequestMethod {
GET, POST, PUT
}
/**
* GETリクエストを出す
* @param {String} endpoint URL
* @param {Map} mapHeader ヘッダー
* @param {Map} mapParam パラメータ
* @return {HttpResponse} レスポンス
*/
public static HttpResponse sendGetReqeust(String endpoint,
Map<String, Object> mapHeader,
Map<String, Object> mapParam) {
return sendRequest(endpoint, RequestMethod.GET, mapHeader, mapParam);
}
/**
* POSTリクエストを出す
* @param {String} endpoint URL
* @param {Map} mapHeader ヘッダー
* @param {Map} mapParam パラメーラ
* @return {HttpResponse} レスポンス
*/
public static HttpResponse sendPostReqeust(String endpoint,
Map<String, Object> mapHeader,
Map<String, Object> mapParam) {
return sendRequest(endpoint, RequestMethod.POST, mapHeader, mapParam);
}
/**
* PUTリクエストを出す
* @param {String} endpoint URL
* @param {Map} mapHeader ヘッダー
* @param {Map} mapParam パラメータ
* @return {HttpResponse} レスポンス
*/
public static HttpResponse sendPutReqeust(String endpoint,
Map<String, Object> mapHeader,
Map<String, Object> mapParam) {
return sendRequest(url, RequestMethod.PUT, mapHeader, mapParam);
}
/**
* リクエストを出す
* @param url endpoint URL
* @param {RequestMethod} method 請求メソッド
* @param {Map} mapHeader ヘッダー
* @param {Map} mapParam パラメータ
* @return {HttpResponse} レスポンス
*/
private static HttpResponse sendRequest(String endpoint, RequestMethod method,
Map<String, Object> mapHeader,
Map<String, Object> mapParam) {
//リクエスト作成
HttpRequest request = new HttpRequest();
if (method.equals(RequestMethod.GET)) {
// パラメータ設定
List <String> paramList = new List <String>();
for (String key : mapParam.keySet()) {
paramList.add(key + '=' + EncodingUtil.urlEncode(String.valueOf(mapParam.get(key)), 'UTF-8'));
}
if (paramList.size() > 0) {
String param = '?' + String.join(paramList, '&');
url += param;
}
} else {
String param = JSON.serialize(mapParam);
request.setBody(param);
}
//ヘッダー設定
for (String key : mapHeader.keySet()) {
request.setHeader(key, (String)mapHeader.get(key));
}
//請求メソッドを設定
request.setMethod(method.name());
//エンドポイント設定
request.setEndpoint(endpoint);
Http http = new Http();
HttpResponse response = http.send(request);
// エラー無しの場合レスポンスを返却
return response;
}
/**
* レスポンスのボディーを取得
* @param {HttpResponse} response 請求メソッド
* @return {String} レスポンスのボディー
*/
public static String processResponse(HttpResponse response) {
if (response.getStatusCode() == 200) {
return response.getBody();
} else {
// エラー処理などを行う場合は追加してください
return null;
}
}
}
参考
Improve Apex REST Callouts Techniques | Salesforce Trailhead
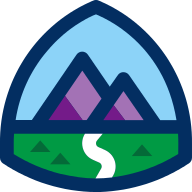
Salesforce Developers
Salesforce Developers