LWC で CSV 出力方法説明
LWC で CSV ファイルを出力する考え方は主に下記の流れとなります。
1.LWC のコンポーネントにデータを持たせます。
2.CSV を作成するため、各行の値""
で囲みます。
3.CSV を作成するため、各行の値は,
で区切します。
4.CSV を作成するため、各行の後ろは\n
で改行します。 5.data:text/csv;charset=utf-8,
+ encodeURI(処理後のデータ
)をリンクの URL を設定します。 6.以上のやり方で CSV を出力できるようにします。
実装方法
- exportDataToCSVInLWC
<template>
<lightning-card
title="Export Data as CSV in Lightning Web Component"
icon-name="custom:custom63"
>
<template if:true="{data}">
<div class="slds-p-around_medium lgc-bg-inverse">
<p>
Do you want download data as a CSV format, Click Here
<lightning-button
icon-name="utility:download"
label="Download as CSV"
title="Download CSV File"
onclick="{downloadCSVFile}"
variant="brand"
></lightning-button>
</p>
</div>
<div class="slds-m-around_medium">
<!-- Datatable component -->
<lightning-datatable
columns="{columns}"
data="{data}"
hide-checkbox-column="true"
key-field="id"
>
</lightning-datatable>
</div>
</template>
</lightning-card>
</template>
import { LightningElement, track } from "lwc";
// importing accounts
import getAccountList from "@salesforce/apex/LWCExampleController.getAccounts";
// imported to show toast messages
import { ShowToastEvent } from "lightning/platformShowToastEvent";
// datatable columns
const cols = [
{ label: "Name", fieldName: "Name" },
{ label: "Industry", fieldName: "Industry" },
{ label: "Type", fieldName: "Type" },
{ label: "Phone", fieldName: "Phone", type: "phone" },
{ label: "Rating", fieldName: "Rating" },
{ label: "Account Number", fieldName: "AccountNumber" },
];
export default class ExportDataToCSVInLWC extends LightningElement {
@track error;
@track data;
@track columns = cols;
// this constructor invoke when component is created.
// once component is created it will fetch the accounts
constructor() {
super();
this.getallaccounts();
}
// fetching accounts from server
getallaccounts() {
getAccountList()
.then((result) => {
this.data = result;
this.error = undefined;
})
.catch((error) => {
this.error = error;
this.dispatchEvent(
new ShowToastEvent({
title: "Error while getting Accounts",
message: error.message,
variant: "error",
})
);
this.data = undefined;
});
}
// this method validates the data and creates the csv file to download
downloadCSVFile() {
let rowEnd = "\n";
let csvString = "";
// this set elminates the duplicates if have any duplicate keys
let rowData = new Set();
// getting keys from data
this.data.forEach(function (record) {
Object.keys(record).forEach(function (key) {
rowData.add(key);
});
});
// Array.from() method returns an Array object from any object with a length property or an iterable object.
rowData = Array.from(rowData);
// splitting using ','
csvString += rowData.join(",");
csvString += rowEnd;
// main for loop to get the data based on key value
for (let i = 0; i < this.data.length; i++) {
let colValue = 0;
// validating keys in data
for (let key in rowData) {
if (rowData.hasOwnProperty(key)) {
// Key value
// Ex: Id, Name
let rowKey = rowData[key];
// add , after every value except the first.
if (colValue > 0) {
csvString += ",";
}
// If the column is undefined, it as blank in the CSV file.
let value =
this.data[i][rowKey] === undefined ? "" : this.data[i][rowKey];
csvString += '"' + value + '"';
colValue++;
}
}
csvString += rowEnd;
}
// Creating anchor element to download
let downloadElement = document.createElement("a");
// This encodeURI encodes special characters, except: , / ? : @ & = + $ # (Use encodeURIComponent() to encode these characters).
downloadElement.href =
"data:text/csv;charset=utf-8," + encodeURI(csvString);
downloadElement.target = "_self";
// CSV File Name
downloadElement.download = "Account Data.csv";
// below statement is required if you are using firefox browser
document.body.appendChild(downloadElement);
// click() Javascript function to download CSV file
downloadElement.click();
}
}
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata" fqn="exportDataToCSVInLWC">
<apiVersion>53.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__Tab</target>
</targets>
</LightningComponentBundle>
動作確認
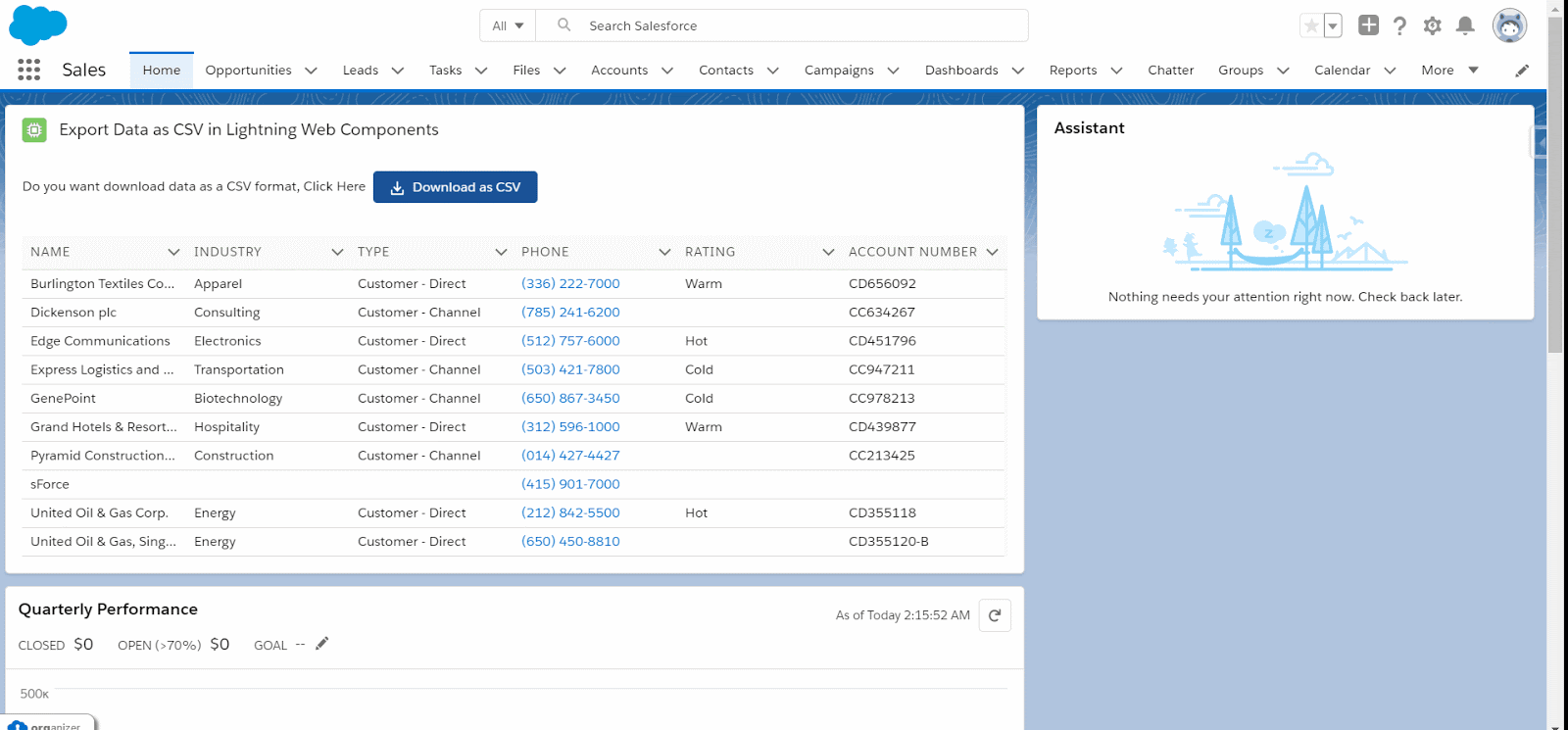